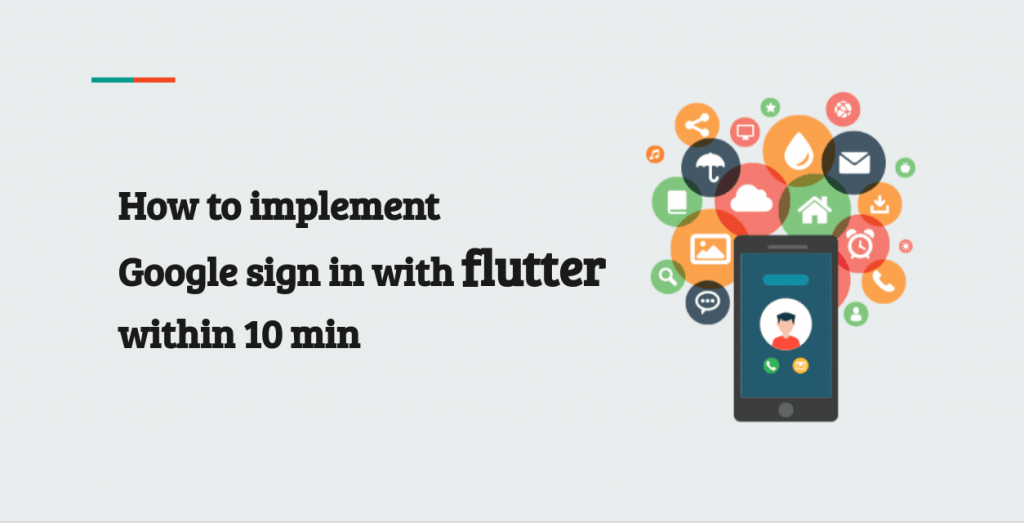
How to implement Google sign in with flutter within 10 min
Hello guys, Welcome to my flutter tutorial series. if don’t know me yet, here is a brief description of me. And before you start If you haven’t read about the new flutter 2.2 features. Please read my article about all the new features of Flutter 2.2
Today I will be talking about how to authenticate your flutter app using flutter firebase auth. But I’ll especially explain the Google sign in with flutter because it is the most commonly used firebase_auth method among other authentication methods.
First, lets start with adding required dependancies to your project
firebase_auth: ^0.16.0
google_sign_in: ^4.4.4
Please make sure that when you are getting these firebase_auth and google_sign_in libraries get the latest one.
Now, we are gonna do some configurations in our firebase console. If you haven’t set up your firebase account yet, log in to firebase with your Gmail account. Once you logged in, then create a project In the firebase console and go inside the project
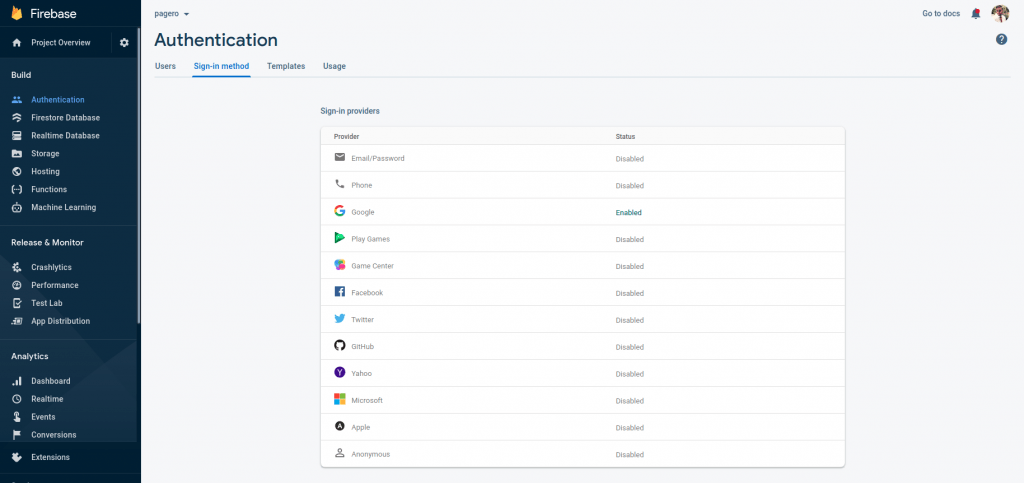
Now you need to go to the authentication tab as it showed in the above images and you need to enable Google authentication from the Sign-in provider’s list. Then you will be asked to follow some steps to enable authentication. Please follow those instructions.
Now let’s start with implementing firebase_auth with our application.
Import firebase_auth and google_sign_in and create instances
import 'package:firebase_auth/firebase_auth.dart';
import 'package:google_sign_in/google_sign_in.dart';
final FirebaseAuth _auth = FirebaseAuth.instance;
final GoogleSignIn googleSignIn = GoogleSignIn();
Sign-in method
Below code will authenticate your user and return the current user
Future signInWithGoogle() async {
final GoogleSignInAccount googleSignInAccount = await googleSignIn.signIn();
final GoogleSignInAuthentication googleSignInAuthentication =
await googleSignInAccount.authentication;
final AuthCredential credential = GoogleAuthProvider.getCredential(
accessToken: googleSignInAuthentication.accessToken,
idToken: googleSignInAuthentication.idToken,
);
final AuthResult authResult = await _auth.signInWithCredential(credential);
final FirebaseUser user = authResult.user;
assert(!user.isAnonymous);
assert(await user.getIdToken() != null);
final FirebaseUser currentUser = await _auth.currentUser();
assert(user.uid == currentUser.uid);
return currentUser;
}
Now, lets implement sign out method
void signOutGoogle() async {
await FirebaseAuth.instance.signOut();
await googleSignIn.signOut();
print("User Sign Out");
}
Now, we have implemented functions to sign in and sign out with google authentication. But we don’t need users to log in whenever they open the application. So, now we are going to get the currently logged-in user.
Future getCurrentUser() async {
FirebaseUser _user = await FirebaseAuth.instance.currentUser();
return _user;
}
With the above code, you can get the currently logged-in user at the splash screen in your app and then you can decide whether to display login UI or main screen. Now we have all the functions that we need to authenticate the user.
This is you can decide whether to display the login screen or not
dynamic result = await getCurrentUser();
if (result != null) {
FirebaseUser user = result;
globals.currentUser = user;
Navigator.pushReplacementNamed(context, '/mainPage');
} else {
Navigator.pushReplacementNamed(context, '/login');
}
Now, I am going to implement a sample login screen with google sign-in flutter
class LoginPage extends StatefulWidget {
@override
_LoginPageState createState() => _LoginPageState();
}
class _LoginPageState extends State<LoginPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
constraints: BoxConstraints.expand(),
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage("images/background.jpg"),
fit: BoxFit.cover)),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Column(
children: <Widget>[
Text("WELCOME TO THE DEMO", style: TextStyle(fontSize: 20.0, fontWeight: FontWeight.w900),),
SizedBox(height: 200.0),
SignInButtonWidget(),
],
),
],
),
),
),
);
}
}
So, guys, this is all you have to do to implement firebase auth with your flutter app. I hope you enjoyed this coding tutorial. If you have any question, please feel free to ask them in the comment section.
If you are interested in learning google authentication with the Spring-boot application, please read about google auth with Spring-boot article.
Follow me on medium ..!!
Don’t forget to rate this article
#customauthentication #Docker #flutter #flutter2.2 #google #googleauthentication #Intellij #java #Javascript #Microservices #mobileappdevelopment #Oauth2 #React.js #React.Memo #ReactHooks #remotedebug #SprintBoot #tomcat #useEffect #useLayoutEffect #weblogic #weblogic12c #WorkFromHome android apps driving-licence DrivingExam flutter learners LearnesEka react.js reacthooks Sri-Lankan Sri-lankan-driving-exam Sri_Lankan useMemo useQuery useTranslations WordPress wordreminder