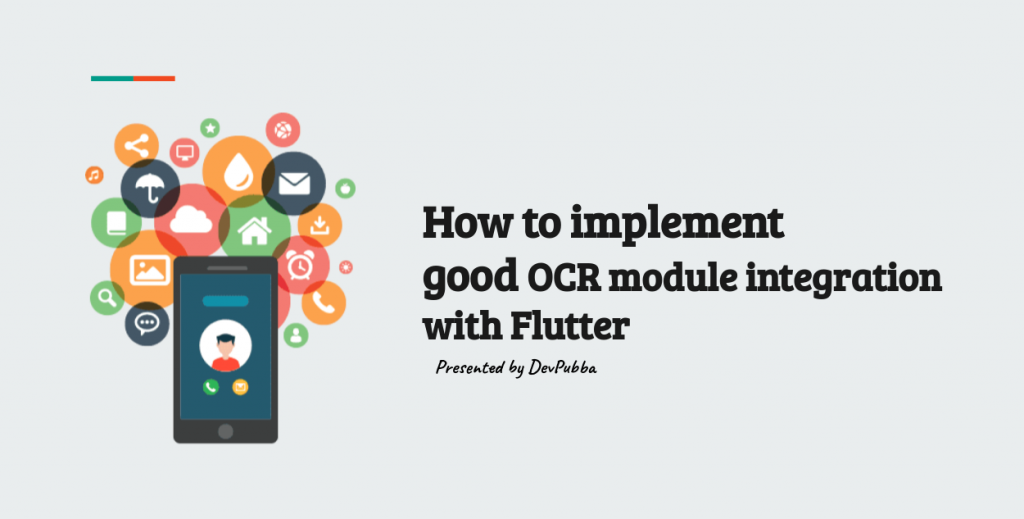
How to implement good OCR module integration with Flutter
This article is focused on implementing an ORC module with your flutter application in order to scan text from somewhere to your application. This is a very simple task with flutter. We are going to use the flutter_mobile_vision library in this tutorial.
The first step is to get the library to your application. In order to do it add this flutter_mobile_vision dependency to your pubspec.yaml file
dependencies: flutter: sdk: flutter flutter_mobile_vision: ^0.1.3
Then the next step is to get permission to access the device camera. In order to get permission add the following code to the AndroidManifest.xml file.
<uses-feature android:name="android.hardware.camera" /> <uses-permission android:name="android.permission.CAMERA" />
Now you have gained the camera permission, but you need to add one more code line to the AndroidManifest.xml file. Add the following code line inside <application> tag like below
<activity android:name="io.github.edufolly.fluttermobilevision.ocr.OcrCaptureActivity" />
Note that the user need to accept the permission in order to access the camera.
Now all the configurations are done and now it is time to do coding. We are going to write the code to open the camera and capture the text. You will be getting the text once you tap on the rectangle box that contains the word or sentence. There are few properties you need to define first, lets get an idea about what are they and what are they doing.
int _cameraOcr = FlutterMobileVision.CAMERA_BACK;
bool _autoFocusOcr = true;
bool _torchOcr = false;
bool _showTextOcr = true;
Size _previewOcr;
You can turn on and off certain features with the above variables. Such as you can decide which camera to use to capture the images. We will be using the above variables in the below method when reading the texts.
Add this code and call it inside initState() method. This will set the _previewOcr variable value.
Future<Null> initialCameraSetup() async {
FlutterMobileVision.start().then((previewSizes) => setState(() {
_previewOcr = previewSizes[_cameraOcr].first;
}));
}
This method will do the reading part from camera
Future_read() async { List texts = []; try { texts = await FlutterMobileVision.read( flash: _torchOcr, autoFocus: _autoFocusOcr, multiple: false, camera: _cameraOcr, waitTap: true, showText: _showTextOcr, preview: _previewOcr, fps: 2.0, ); } on Exception { texts.add(OcrText('Failed to recognize text.')); } if (!mounted) return; setState( () => word = texts[0].value); }
https://play.google.com/store/apps/details?id=com.devpubba.wordreminder&hl=enYou can access the scanned texts from the array and do whatever you want to do with it. This is very simple as I mentioned above. I have used this library in my word reminder app
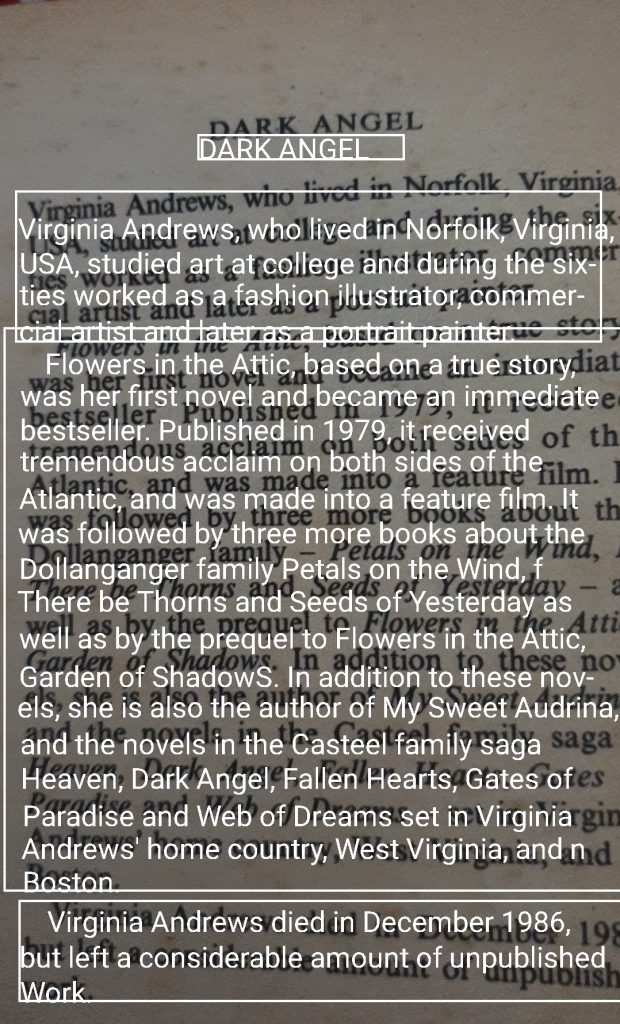
You can read more with following link: https://pub.dev/packages/flutter_mobile_vision#-readme-tab-
Thank you ..! 🙂