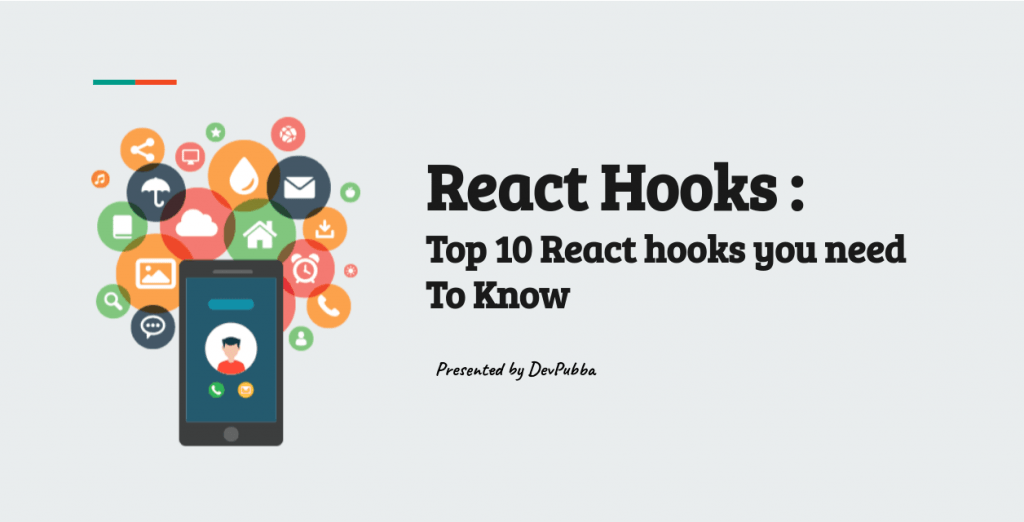
React hooks | Top 10 React hooks you need to know
Today, we will learn about the top 10 React hooks you can use when developing your application. And today, We aren’t going to talk about useEffect, useLayoutEffect, and useState hooks. Because everyone is talking about them. Let’s talks about something else today. However, if you like you can read about What is the real difference between React useEffect and useLayoutEffect?
First of all, If you don’t know who I am, please visit my personal blog to read about me and my other articles.
1. useMemo from React Hooks
This React hook will improve the performance of your website, having optimised computational cost when you are performing expensive calculations. React memo will memorize return values, and it will only operate when provides dependencies are changed. If you don’t have much idea about how React memo works. Please read my article about Why React.memo is important?
const value = useMemo(
() =>
// This part will only be executed when one of given dependencies is changed
options.find(
({ value }) =>
value.startDate === startDate && value.endDate === endDate
) ?? customDateRangeOption,
// Dependancy array
[startDate, options, endDate]
);
2. useCallback from React Hooks
Previously, We talked about memorizing values with useMemo. The difference between useMemo and useCallback is that useCallback memorize the whole function.
const memoizedCallback = useCallback(
() => {
someFuntion(a, b);
},
[a, b],
);
3. useRef from React Hooks
This React hook will allow us to create mutable reference objects in which the .current property is initialized to the passed argument. This returned object will persist for the full lifetime of the component. Most importantly, even though the component re-render this ref value will not be changed.
const btnRef = useRef(null);
return (
<button onClick={onButtonClick} ref={btnRef}>Accepted</button>
)
4. useTranslation from React-i8next
The react-i8next library provides this React hook. With this hook, you can easily add translation support to your website. Let me show an example
import {useTranslation} from "react-i18next";
export MyComponent = () => {
const {t} = useTranslation();
return <p>{t('my translated text')}</p>
}
5. useParams from React-router
This React hook comes from the react-router library. This returns an object of key-value pairs of URL parameters. For example, Let’s say your website URL is like this:
localhost:3000/sampleapp/inbox?name=pubudu
and
In addition to that our router path is like this
<Route path="/sampleapp/:status">
After that, if we query status with the useParams as below, you will get status as inbox
function BlogPost() {
let { status } = useParams();
return <div>Now showing post {status}</div>;
}
6. useLocation from React-router
The useLocation
hook returns the location
object that represents the current URL. You can think about it like a useState
that returns a new location
whenever the URL changes.
let location = useLocation();
This useLocation and useParams React hooks comes from react-router. Please read their official documentation.
7. useMediaQuery from React-Responsive
In order to, develop responsive web applications, you need to know media queries. To make your life simple now, there is a hook we can use for media queries.
import React from 'react';
import useMediaQuery from '@material-ui/core/useMediaQuery';
export default function SimpleMediaQuery() {
const matches = useMediaQuery('(min-width:600px)');
return <span>{`(min-width:600px) matches: ${matches}`}</span>;
}
8. useQuery from React-Query
Now, we are going to talk about the useQuery hook from React query library. React query library is often described as the missing data-fetching library for React. Still, it makes fetching, caching, synchronizing, and updating server state in your React applications a breeze in more technical terms.
import { useQuery } from 'react-query'
function App() {
const { isLoading, isError, data, error } = useQuery('todos', fetchTodoList)
}
However, There are a lot more thing you can do with react-query. Please read their official documentation here
9. useTable from React Table
This is one of the commonly used hooks within developers trying to populate a table in their web apps. Most importantly, React-table has lots of hooks that you can use to reduce your development time.
//Use the state and functions returned from useTable to build your UI
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable({columns,data})
When you feed the useTable hook with your columns and data, the hook will provide you with several functions and props to build your UI with them. React-table is a perfect library. Please read their official documentation here.
10. useDebugValue
useDebugValue
from React hooks can be used to display a label for custom hooks in React DevTools. However, it is not recommended to add debug values to every custom Hook
function useFriendStatus(friendID) {
const [isOnline, setIsOnline] = useState(null);
useDebugValue(isOnline ? 'Online' : 'Offline');
return isOnline;
}
Finally, these are the react hooks that I wanted to talk about with you today. However, Today we didn’t talk much about commonly used react hooks because you might already know them. In short, today, I wanted to introduce to you a few uncommon hooks that you can use with your development. The React-table, React-responsive, and React-query libraries will be very valuable for you. I have used all of these libraries with my development. Therefore I know that those are really helpful.
Let us know your opinion about this article so we can further improve our writings, and if you have any question please ask them in the comment section.
After all, Thank you for your valuable time and however if you like this article, please share this with your colleagues. 🙂
#customauthentication #Docker #flutter #flutter2.2 #google #googleauthentication #Intellij #java #Javascript #Microservices #mobileappdevelopment #Oauth2 #React.js #React.Memo #ReactHooks #remotedebug #SprintBoot #tomcat #useEffect #useLayoutEffect #weblogic #weblogic12c #WorkFromHome android apps driving-licence DrivingExam flutter learners LearnesEka react.js reacthooks Sri-Lankan Sri-lankan-driving-exam Sri_Lankan useMemo useQuery useTranslations WordPress wordreminder